Imagine you’re a recruiter with a stack of resumes to process…
Each one contains critical details—skills, experience, contact information—that you need to organize into a database. Doing this by hand is slow, error-prone, and honestly, a bit of a drag. What if you could automate it?
With OpenAI’s Function Calling feature, you can teach an AI to extract exactly what you need from those resumes and deliver it in a tidy, structured format, ready to plug into a database.
In this article, we’ll walk through how to use OpenAI’s Function Calling to parse resumes and extract job applicant details like skills, experience, and contact info into JSON format—a standard that’s perfect for databases. Whether you’re new to AI or just curious about automating repetitive tasks, this tutorial is designed to be simple, practical, and engaging.
By the end, you’ll have a working script, plus links to my GitHub repo and a YouTube video where you can follow along step-by-step.
Let’s dive in!
Introduction to OpenAI's Function Calling
The Problem: AI’s Struggle with Consistency
Before Function Calling came along, getting structured data out of an AI model like ChatGPT was tricky. Imagine asking an AI to list skills from a resume. One time, it might give you a neat list: “Python, Java, SQL.”
Another time, it might ramble: “The applicant knows Python and has worked with Java, oh, and maybe SQL too.”
This inconsistency made automation a headache—how do you reliably feed that into a database?
The Solution: Function Calling
Function Calling changes the game. It’s like giving the AI a clear checklist: “Extract the name, skills, and email, and format it this way.” The AI follows your instructions precisely, delivering consistent, structured output every time.
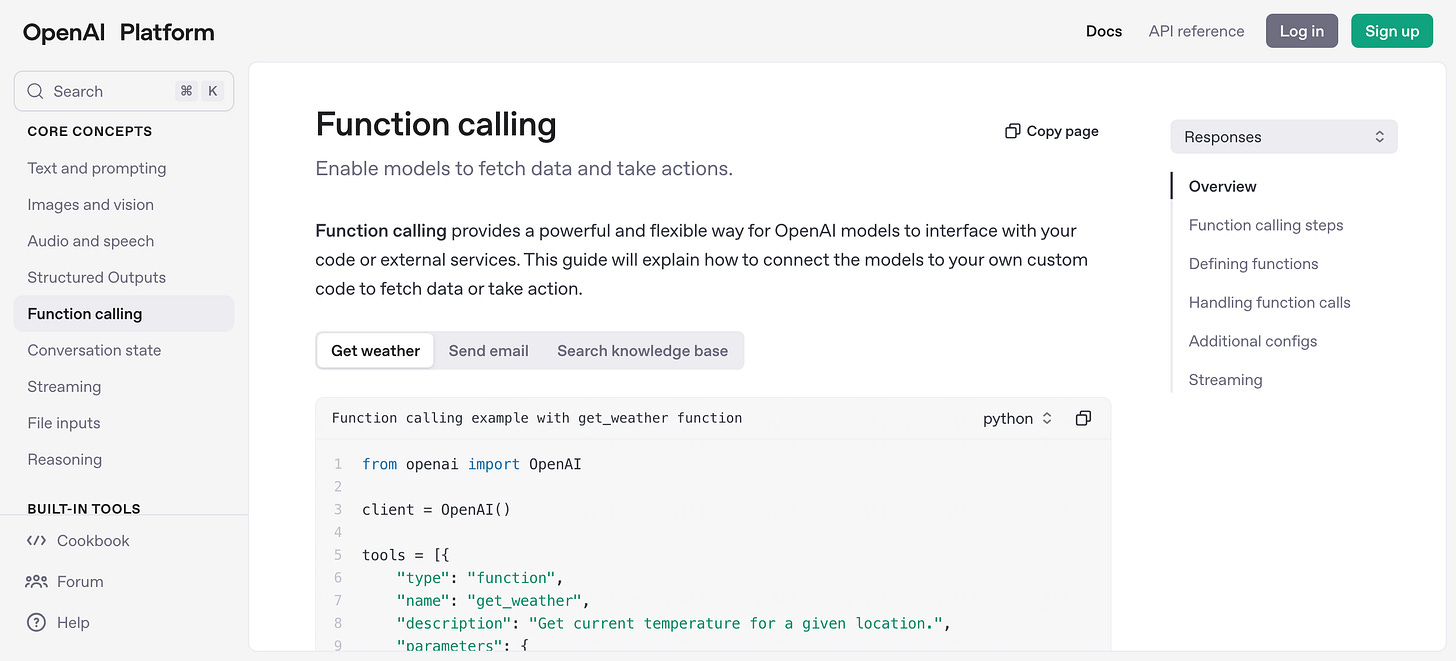
Why JSON?
We’ll use JSON (JavaScript Object Notation) for this structured output.
Why? It’s lightweight, easy to read, and widely supported by databases and programming languages.
For example, instead of a messy text blob, you get something like this:
{
"name": "Jane Smith",
"skills": ["Python", "Data Analysis"],
"email": "jane.smith@example.com"
}
This format is perfect for automation, and we’ll see how to make it happen.
How Function Calling Works in OpenAI APIs
What Is Function Calling?
Think of Function Calling as a way to give the AI a job description. You define a “function” that specifies what data to extract (like skills or contact info) and how to structure it (in JSON). The AI then executes that job and hands you the results.
Here’s a quick example. Suppose you have this text:
“Jane Smith, a data analyst with 3 years of experience, knows Python and SQL. Contact her at jane.smith@example.com.”
With Function Calling, you can tell the AI to extract the name, skills, and email, and it’ll return:
{
"name": "Jane Smith",
"skills": ["Python", "SQL"],
"email": "jane.smith@example.com"
}
Setting Up the API
To make this work, we’ll use OpenAI’s API in Python. You’ll define a function in your code, send it to the API along with the resume text, and get back a structured response. Don’t worry—we’ll walk through every step.
Implementing JSON Data Extraction with OpenAI
Setting Up Your Environment
Before we code, let’s get ready:
Python: Make sure it’s installed (version 3.6 or higher works fine).
OpenAI API Key: Sign up at OpenAI to get one.
Libraries: Install the
openai
library by running this in your terminal:4
pip install openai
That’s it! You’re set to start coding.
Writing Your First Function Call
Let’s create a Python script to extract applicant details from a resume. We’ll focus on name, email, skills, and years of experience.
Create a file called resume_parser.py
and add this code:
import openai
import json
openai.api_key = "your-api-key-here"
def extract_applicant_details(resume_text):
response = openai.chat.completions.create(
model="gpt-4o",
messages=[
{"role": "system",
"content": "You are a resume-parsing assistant."},
{"role": "user",
"content": f"Extract the name, email, skills,
and years of experience from this resume:\n\n
{resume_text}"}
],
functions=[
{
"name": "extract_details",
"description": "Extract applicant details from a resume",
"parameters": {
"type": "object",
"properties": {
"name": {"type": "string"},
"email": {"type": "string"},
"skills": {"type": "array", "items": {"type": "string"}},
"experience_years": {"type": "number"}
},
"required": ["name", "email", "skills", "experience_years"]
}
}
],
function_call={"name": "extract_details"}
)
return json.loads(response.choices[0].message.function_call.arguments)
# Test it with a sample resume
resume = """
Jane Smith
Email: jane.smith@example.com
Skills: Python, SQL, Data Analysis
Experience: 3 years as a Data Analyst
"""
details = extract_applicant_details(resume)
print(json.dumps(details, indent=2))
Run this with python resume_parser.py
, and you should see:
{
"name": "Jane Smith",
"email": "jane.smith@example.com",
"skills": ["Python", "SQL", "Data Analysis"],
"experience_years": 3
}
How It Works
API Key: Replace "
your-api-key-here
" with your actual key.Function Definition: The
functions
list tells OpenAI what data to extract and its format (e.g.,skills
as an array of strings).Messages: The
system
message sets the AI’s role, and theuser
message provides the resume text.Response: The AI returns the data as a JSON string, which we parse with
json.loads
.
Real-World Applications for Developers
Resume parsing is just the beginning. Here’s how Function Calling can level up your projects:
Email Processing: Extract order details or customer info from emails.
Report Analysis: Pull key stats from financial or performance reports.
ETL Pipelines: Build automated systems to extract, transform, and load data into databases.
Imagine automating an entire hiring pipeline—parsing resumes, ranking candidates by skills, and updating a dashboard—all with a few lines of code!
OpenAI’s Function Calling makes it easy to turn chaotic text into structured, actionable data.
Conclusion: Future of Structured AI Outputs
OpenAI’s Function Calling makes it easy to turn chaotic text into structured, actionable data. As AI evolves, tools like this will power smarter automation, from personal projects to enterprise workflows. You’ve now got the basics—why not experiment with your own data?
For more, check out:
My GitHub repo with the full code and sample resumes.
My YouTube tutorial for a step-by-step walkthrough.
Happy coding, and let me know how you use Function Calling in your next project!
🥂🔥🔥🔥
Informative write up